lab13-TextureShadedGeometry
Texture2D 클래스 사용한 Texture Mapping 정의
GeometryPositionNormalTexture 클래스 사용
‘g’-key 사용, Texture Pyramid->Cube->Sphere->Cylinder->Torus->Parallelepiped->Grid 변환
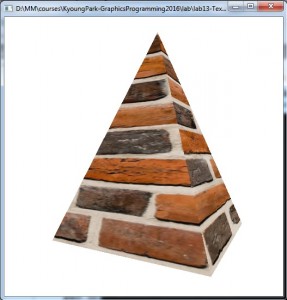
void Cone::init()
{
numVertices = 0;
glm::vec3 n(0.0f, 0.0f, 0.0f);
float xTexCoord = 0.0f;
float dslice = 1.0f / slices;
float theta = (float)(2 * M_PI / slices);
for (int i = 0; i < slices; i++)
{
glm::vec3 v1, v2, v3;
// V1
v1[0] = p[0];
v1[1] = p[1] + height;
v1[2] = p[2];
// V2
v2[0] = p[0] + radius * cosf(theta * i);
v2[1] = p[1];
v2[2] = p[2] + radius * sinf(theta * i);
// V3
v3[0] = p[0] + radius * cosf(theta * ((i + 1) % slices));
v3[1] = p[1];
v3[2] = p[2] + radius * sinf(theta * ((i + 1) % slices));
// Normal
glm::vec3 n = glm::cross((v2-v1), (v3-v1));
n = glm::normalize(n);
printf(“V1: %f %f %f\n”, v1[0], v1[1], v1[2]);
printf(“V2: %f %f %f\n”, v2[0], v2[1], v2[2]);
printf(“V3: %f %f %f\n”, v3[0], v3[1], v3[2]);
printf(“N: %f %f %f\n\n”, n[0], n[1], n[2]);
// V1
vbo.addData(&v1, sizeof(glm::vec3)); // vertex position
vbo.addData(&glm::vec2(xTexCoord + dslice * 0.5f, 1.0f), sizeof(glm::vec2)); // vertex texture coordnate (TopCenter)
vbo.addData(&n, sizeof(glm::vec3)); // vertex normal
// V2
vbo.addData(&v2, sizeof(glm::vec3)); // vertex position
vbo.addData(&glm::vec2(xTexCoord, 0.0f), sizeof(glm::vec2)); // vertex texture coordnate
vbo.addData(&n, sizeof(glm::vec3)); // vertex normal
// V3
vbo.addData(&v3, sizeof(glm::vec3)); // vertex position
vbo.addData(&glm::vec2(xTexCoord + dslice, 0.0f), sizeof(glm::vec2)); // vertex texture coordnate
vbo.addData(&n, sizeof(glm::vec3)); // vertex normal
xTexCoord += dslice; // increment texCoord
numVertices += 3;
}
createVAO();
isLoaded = true;
}