단국대학교 멀티미디어공학전공 HCI프로그래밍2 (2015년 가을학기) 실습
날짜: 2015년 11월 20일
– 실습번호 : lab-04 (Due by 12/11)
– 실습제목 : Window.Forms Controls (ListView, ComboBox, etc), RSS, XML, GMap.Net, YahooWeather, LINQ
– 실습요약 : 지도 위에 현재의 날씨 정보를 보여준다.
– 실습문제
Resources.zip 파일을 사용하여 imageList 를 만든다.
- GMap.Net 컨트롤을 설치한다.
http://www.independent-software.com/gmap-net-tutorial-maps-markers-and-polygons/
http://dis.dankook.ac.kr/lectures/hci15/?p=541 커스텀 컨트롤 추가 참고
private void Form1_Load(object sender, EventArgs e)
{
// Initialize map:
gMapControl1.MapProvider = GMap.NET.MapProviders.GoogleMapProvider.Instance;
GMap.NET.GMaps.Instance.Mode = GMap.NET.AccessMode.ServerOnly;
gMapControl1.SetPositionByKeywords(“Seoul, Korea”);
gMapControl1.CanDragMap = true;
gMapControl1.DragButton = MouseButtons.Left;
gMapControl1.MouseWheelZoomType = GMap.NET.MouseWheelZoomType.MousePositionAndCenter;
gMapControl1.MinZoom = 3;
gMapControl1.MaxZoom = 19;
gMapControl1.Zoom = 4;
gMapControl1.ScrollControlIntoView(this);
// Update gMapMarker using weather list
UpdateWeatherMarker(weatherList); // 구현 필요 – GMapMarkerImage과 GMapMarkerCircle를 사용하여 gMapControl1에 마커를 표현
}
- 야후 날씨 정보 Yahoo Weather RSS Feed 설명
https://developer.yahoo.com/weather/documentation.html
- WOEID (World On Earth ID) 설명
https://developer.yahoo.com/geo/geoplanet/guide/concepts.html
- WOEID.csv 파일을 읽어서 List<WOEID>를 만든다.
NEWWOEID
http://dis.dankook.ac.kr/lectures/hci15/?p=572 Person.csv 파일 읽기 참고
- WOEID (World On Earth ID)에 따라 최근 야후 날씨 정보를 Feed 할 수 있다.
string url = string.Format(“http://weather.yahooapis.com/forecastrss?w={0}”, woeid);
http://weather.yahooapis.com/forecastrss?w=1132599 Seoul Yahoo Weather RSS
Yahoo! Weather – Seoul, KR (Seoul Yahoo Weather RSS XML 파일 예시)
- WeatherData 클래스를 만든다.
public class Condition
{
public string Text { get; set; }
public int Code { get; set; } // 0 (tornado) 3200 (not available)
public int Temperature { get; set; } // cloudy, rain, etc
public string Date { get; set; }
public Color TemperatureColor {} // Fahrenheit Color 계산 구현요망
}
public class Forecast
{
public string Day { get; set; }
public string Date { get; set; }
public int Low { get; set; }
public int High { get; set; }
public string Text { get; set; }
public int Code { get; set; }
}
public class WeatherData
{
public long WOEID { get; set; } // LINQ join을 위해 추가
public string Title { get; set; }
public double Lat { get; set; }
public double Lon { get; set; }
public string Link { get; set; }
public Condition Condition = new Condition();
public List<Forecast> FiveForecast = new List<Forecast>();
}
- List<WOEID>를 이용해서 Rss 피드해서 List<WeatherData >를 만든다.
http://dis.dankook.ac.kr/lectures/hci13/2013/11/26/%ec%a2%85%eb%a1%9c%ea%b5%ac-%ea%b0%80%ed%9a%8c%eb%8f%99-%eb%8f%99%eb%84%a4%ec%98%88%eb%b3%b4-rss-feed/ 기상청 RSS 서비스 Parsing 예시
http://dis.dankook.ac.kr/lectures/hci12/2012/11/21/readrssfeed/ 실시간 지진 데이타 RSS Parsing 예시
- List<WeatherData>를 이용해서 GMap.NET에 marker에는 현재 날씨 상태(Code)에 따른 아이콘 이미지를 보여주고, 그 위에 현재 날씨 온도(Temperature)에 따른 색깔로 원(circle polygon)을 그려준다.
http://www.independent-software.com/gmap-net-tutorial-maps-markers-and-polygons/
class GMapMarkerCircle : GMapMarker { // 날씨 온도에 따른 색깔로 Circle을 그려주고 그 아래엔 온도를 텍스트로 보여줌
public Color MarkerColor { get; set; }
public Brush Fill = null;
// 생성자에서 Fill = new SolidBrush(MarkerColor);
public override void OnRender(Graphics g) {
g.FillEllipse(Fill, new Rectangle(LocalPosition.X – R/2, LocalPosition.Y – R/2, R, R));
}
}
class GMapMarkerImage : GMapMarker { // 날씨 상태에 따른 Image와 Tooltip으로 날씨 상태 정보를 보여줌
public Image MarkerImage { get; set; }
public override void OnRender(Graphics g) {
g.DrawImage(MarkerImage, LocalPosition.X, LocalPosition.Y, Size.Width, Size.Height);
}
}
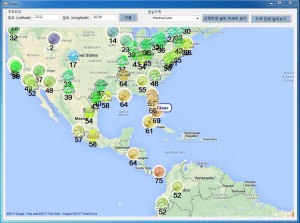
지도에서 아이콘 이미지를 오른쪽 마우스로 클릭하면 위도, 경도 정보가 텍스트박스에 나타남.
private void gMapControl1_OnMarkerClick(GMapMarker item, MouseEventArgs e) { // 내부 구현 필요
}
콤보박스(ComboBox)에서 관심 지역을 선택하면 위도, 경도 정보가 텍스트박스에 나타나고 해당 지역으로 이동함.
private void comboBox1_SelectedValueChanged(object sender, EventArgs e) { // 내부 구현 필요
}
위도 경도 정보를 넣고 ‘이동’ 버튼을 누르면 해당 지역으로 이동함.
private void button1_Click(object sender, EventArgs e) { // 내부구현 필요
}
‘선택지역 날씨 자세히 보기’ 버튼을 누르면 웹 페이지를 띄움.
private void button2_Click(object sender, EventArgs e) { // 내부구현 필요
}
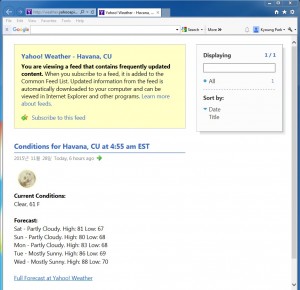
‘지역 전체 날씨보기’ 버튼을 누르면 비모달형 Form2가 뜨면서 지도에 보이는 지역의 날씨 정보를 ListView로 보여줌.
private void button3_Click(object sender, EventArgs e) { // 내부구현 필요
}
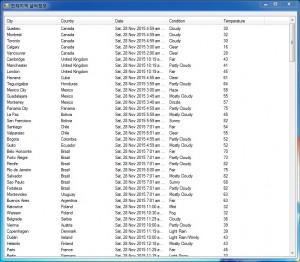
private void Form2_Load(object sender, EventArgs e) { // 내부구현 필요
// LINQ Joined Query 를 통하여 listView item에 추가
}